python class decorator inheritance
Selfdata data def add_one Stack Exchange Network Stack Exchange network consists of 179 QA communities including Stack Overflow the largest most trusted online community for developers to learn share their knowledge and build their. Decorators should be preferred when you dont have an is-a relationship.
Beginner S Guide To Abstract Base Class In Python Dev Community
Introductory topics in object-oriented programming in Python and more generally include things like defining classes creating objects instance variables the basics of inheritance and maybe even some special methods like __str__.
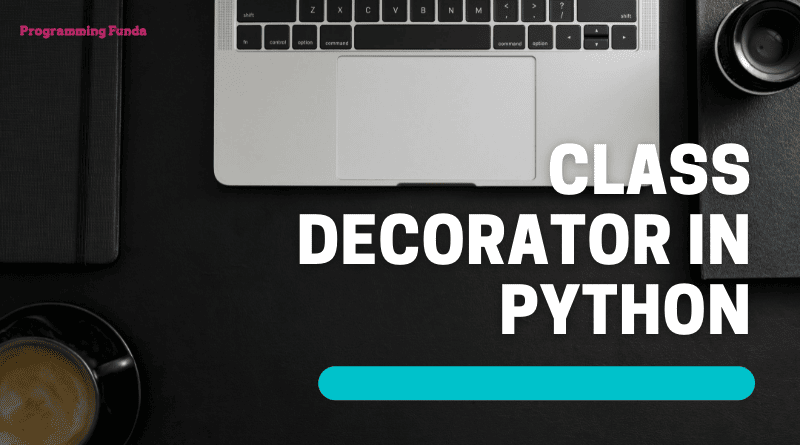
. Return func return inner. Decorators are a very powerful and useful tool in Python since it allows programmers to modify the behaviour of function or class. In both cases decorating adds functionality to existing functions.
From there you can access the iterations variable. Parent class is the class being inherited from also called base class. Decorators are a very powerful and useful tool in Python since it allows programmers to modify the behaviour of function or class.
A polygon is a closed figure with 3 or more sides. The star decorator factory takes an argument and returns a callable. Python python So by using the property decorator you can simplify the property definition for a class.
When a child class inherits from only one parent class it is called single inheritance. Inheritance is a powerful feature of OOP that allows programmers to enable a new class to receive - or inherit all the properties methods of existing classclasses. Python decorators vs inheritance.
Say we have a class called Polygon defined as follows. Therefore you can make the __call__ method as a decorator. Lets learn how to use it.
Decorators allow us to wrap another function in order to extend the behaviour of the wrapped function without permanently modifying it. Inheriting decorating functions in Python. The following adds a setter method set_age to assign a value to _age attribute to the Person class.
Example of Inheritance in Python. I found a solution to your problem. Def decorator iterations source.
Those decorators have similarities to inheritance between classes. This class has data attributes to store the number of sides n and magnitude of each side as a list called sides. Class Inheritance allows to create classes based on other classes with the aim of reusing Python code that has already been implemented instead of having to reimplement similar code.
Python decorators and class inheritance. We can add functionality to the function by defining methods in the decorating class. How can i calculate the time taken by an overridden method in python with just a methoddecorator in base class.
In Python the classmethod decorator is used to declare a method in the class as a class method that can be called using ClassNameMethodNameThe class method can also be called using an object of the class. Prefer composition over inheritance. Decorators in Python.
What are metaclasses in Python. Inheritance allows us to define a class that inherits all the methods and properties from another class. Since that decorator is a function wrapping your method it has access to the class instance using the args first index.
When the dataclass is being created by the dataclass decorator it looks through all of the classs base classes in reverse MRO that is starting at object and for each dataclass that it finds adds the fields from that base class to an ordered mapping of fieldsAfter all of the base class fields are added it adds its own fields to the ordered mapping. The idea is to write your own decorator. I will review pros and cons for class-inheritance and decorator functionclass based.
You can use decorators to change classes in a way inheritance would do. These can of course be. When a child class inherits from multiple parent classes it is called multiple inheritance.
Unlike java python shows multiple inheritance. Inheritance abstraction encapsulation and polymorphism are the four fundamental concepts provided by OOP Object Oriented Programming. See the PEP for why this was added to PythonSee also PEP 3141 and the numbers module regarding a type hierarchy for numbers based on ABCs.
Because Data Classes use normal class definition syntax you are free to use inheritance metaclasses docstrings user-defined methods class factories and other Python class features. My goal was to be able to have an object with methods. Python Class Method Decorator classmethod.
A class decorator is provided which inspects a class definition for variables with type annotations as defined in PEP 526 Syntax for Variable Annotations. To demonstrate the use of inheritance let us take an example. In Python decorators can be either functions or classes.
It is recommended to use the. The classmethod is an alternative of the classmethod function. For example a decorator that propagates documentation from another class or registers a class in some collection.
Decoration typically only affects the class it decorates but not classes that inherit from the base class. We can define a decorator as a class in order to do that we have to use a. The collections module has some concrete classes that derive from ABCs.
If this is a good choice or not heavily depends on the architecture of your Python project as a whole. Python is an object oriented programming language though it doesnt support strong encapsulation. Child class is the class that inherits from another class also called derived class.
We saw an example above. When you create a Python application there is one thing that can make your life a lot easier. The standard librarys dataclass decorator is an excellent example of a sensible usage choosing decorators over inheritance.
When we decorate a function with a class that function becomes an instance of the class. This module provides the infrastructure for defining abstract base classes ABCs in Python as outlined in PEP 3119. In previous article about class-based decorators for classes I gave you example how one can create decorator.
Decorators allow us to wrap another function in order to extend the behaviour of the wrapped function without permanently modifying it. The callable takes an argument fn which is a function that will be decoratedAlso the callable can access the argument n passed to the decorator factoryA class instance can be a callable when it implements the __call__ method. Well discuss that in a second.
Abstract Factory Pattern In Java Pattern Sequence Diagram Pattern Design
Build Factory And Utility In Your Python Classes By Christopher Tao Towards Data Science
How To Use Class Decorator In Python
Decorator Design Pattern In Java With Example Java67 Design Patterns In Java Pattern Design Java Tutorial
What You Probably Don T Know About Python Decorators Hackernoon
Inheritance In Python Episode 14 Invent Your Shit
Private Method Without Underscores And Interfaces In Python By Dmytro Striletskyi Medium
Python Classes And Objects Object Oriented Programming Edureka
Delivery Packages In Odoo 13 Economic Environment Research Scholar Rounding Strategies
Class Attributes And Methods Learn Programming Method Class
Oop Using Inheritance To Provide One Class By Another In Python Stack Overflow
Implementing The Decorator Pattern In Python Dev Community
This Keyword In Java This Keyword In Java Is A Reference Variable That Refers To The Current Class Object In Other Words It H Java Tutorial Java Keywords
Python Combine Multiple Class Decorators And Get Same Behaviour In Pycharm Stack Overflow
Model Constraints Python Constraints In Odoo Python Technical Video Messages
Python Belajar Classmethod Jago Ngoding
What Is Decorator Design Pattern
Tutorial Python Classes Learn Web Tutorials
Flyweight Design Pattern Design Pattern Java Pattern Design Pattern